Binary Thresholding Using OpenCV
What is Binary Thresholding?
Threshloding in general is an Image pre-proccessing Technique that is used to to filter out certain color in an image, where Binary Thresholding is used to filter a grey scale color.
So if we threshold at a value of 40, assuming that a grey scale image single pixel is in the range of (0-255), that means that all values that are higher than 40, will be 0.
cv2.threshold :
In OpenCV we use the function cv2.thrshold to perform this operation.
cv2. threshold (src, thresh, maxValue, cv2. THRESH BINARY)
cv2.threshold Arguments:
- src: This is used to specify the input image. It should be a grey scale image in our case.
- thresh: This is our filter value. Meaning all value up to thresh will be kept as it is.
- maxValue: Here we specify the value that will replace all values exceeding thresh. In our example, all values that are higher than 40 will be replaced with this parameter.
- cv2. THRESH BINARY: Which is the filter we will be using. In our case, this is a binary filter. There are other arguments for other filters as well.
Binary Threshold Example:
Let's assume that we have Bottle labeling machine,And we would like to filter out the bottle we have in order to process it further.
Let's say that this is what our camera has captured:
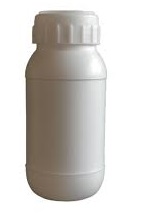
This is what our code would look like:
#import opency
import cv2
# Read the image
src= cv2.imread("bottle.jpg")
# Set the threshold and maxValue
thresh = 245
maxValue = 255
# Basic threshold example
th, dst = cv2.threshold (src, thresh, maxValue, cv2. THRESH_BINARY)
#Save the image
cv2.imwrite ("pic threshold.jpg",dst)
The result would look something like that:
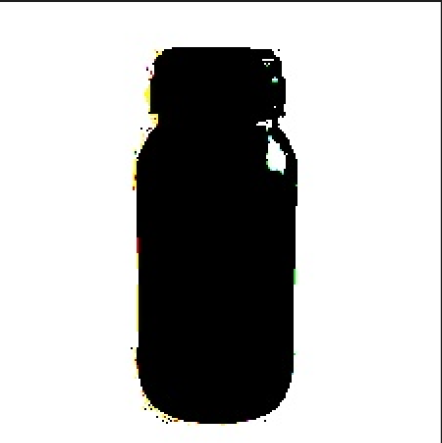
What we have done now, is that we have roughly seperated the bottle from the background.
In the Next Tutorial We will learn how to contour our object to prepare it for further processing.
Check it out.