Arrays using Structured Text - IEC-61131-3
Why use arrays in the first place?
Consider that you have 3 variables of each of the following types:
- INT
- REAL
- BOOL
The trivial way to declare those variable would be like this:
Structured text supports direct Arithmetic Operations like Addition, Subtraction, Division and Muliplication:
//structured Text Multiple Variable Declaration
x1: bool;
x2: bool;
x3: bool;
y1: int;
y2: int;
y3: int;
z1: bool;
z2: bool;
z3: bool;
Well that is a lot of variables, and with larger codes, this will get messy.
Here is where Arrays jump in to clean up the code
//Structured Text Array Declaration
myArrayINT: ARRAY[0..2] OF INT;
myArrayREAL: ARRAY[0..2] OF REAL;
myArrayBOOL: ARRAY[0..2] OF BOOL;
We first choose the name of our array, like myArrayINT,
then we add ARRAY and both an upper and a lower limit.
We choose the array size. In our case it will contain 3 elements, with indexes 0,1,2
Then we specifiy what type will this array hold, in our example we have INT, REAL , BOOL
myArrayINT : Name of the array.
ARRAY[0..2] : Array size, or upper/lower limits.
OF INT : Array type.
Accessing and modifiying Structured Text Array Elements
Accessing elements is done by specifiying the name of the array and adding the index in brackets.
Notice the minimum index is the lower limit we specified which is 0, and the same goes for the upper limit which is 2
//Structured Text Accessing Array Elements
myArrayINT[0]:=15;
myArrayINT[1]:=11;
myArrayINT[2]:=18;
myArrayREAL[0]:=7.2;
myArrayREAL[1]:=178.6;
myArrayREAL[2]:=1.18;
myArrayBOOL[0]:=1;
myArrayBOOL[1]:=0;
myArrayBOOL[2]:=1;
Arrays and FOR loops
The most important use of arrays comes along with FOR loops, which allow itiration across array elements
Example:
Assume that we have one Button to turn on 10 devices, assume motors.
by simply creating an array of 10 motors, and one button variable, we can achieve this easily.
//Structured Text Declaration
i:int:=0;
myArrayOfAllMotors: ARRAY[0..9] OF bool;
oneButtonTurnAllOnOrOFF: bool;
//Structured Text Arrays and Loops
IF oneButtonTurnAllOn = 1 THEN
FOR i := 0 TO 9 DO
myArrayOfAllMotors[i]:= TRUE;
END_FOR
What we are saying is, if that general one button is pressed,
then start a FOR loop itirating all motors from 0 to 9
and for every i ,in myArrayOfAllMotors[i], turn on the motor.
Since as you know, the i will sweep values from 0 to 9 scanning all motors.
Let us add ELSE to turn OFF all motors
By adding an ELSE we can now turn off motors
when the button is OFF.
//Structured Text Arrays and Loops
IF oneButtonTurnAllOn = 1 THEN
FOR i := 0 TO 9 DO
myArrayOfAllMotors[i]:= true;
END_FOR
ELSE
FOR i := 0 TO 9 DO
myArrayOfAllMotors[i]:= false;
END_FOR
END_IF
Demo
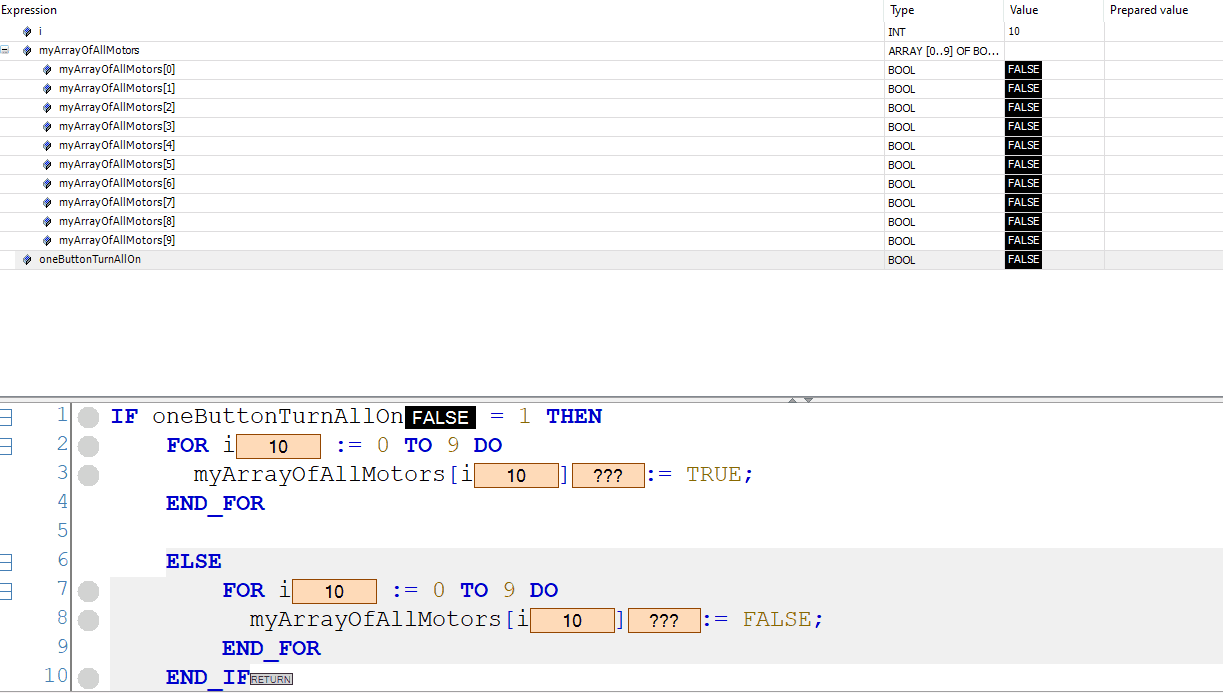