Automated Form filling using Selenium Python 2022
In this tutorial we are going to create an automated script to fillout online forms using python. We will setup selenium and learn how to use it to automate the process. Lets get started!
Setting up selenium-wireusing pip
First use pip command to install selenium-wire package.
pip install selenium-wire
Installing Chrome Driver
Before we can do any web automation, we need to install Chrome Virtual Driver.
Just go to google and seatch for ChromDriver:
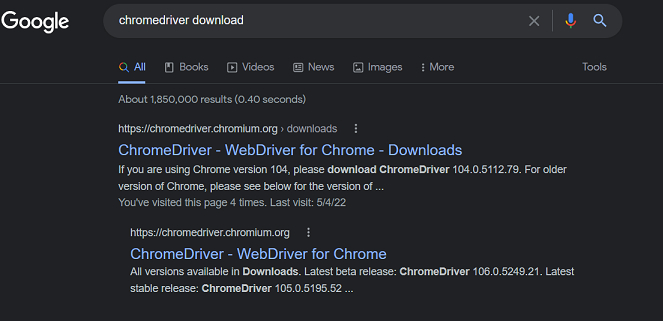
Install the vwindows version, latest stable would do just fine:
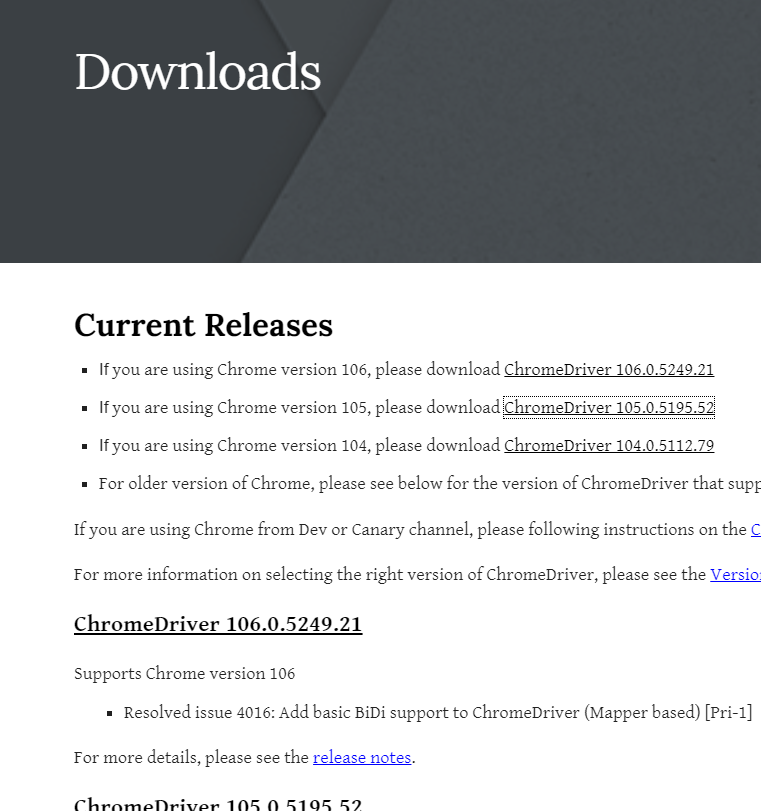
Testing if Chrome Driver is working:
Copy the downloaded file to your python script directory.
Import the libraries, and define our Webdriver, and you should get a new browser window opened
from time import sleep
from seleniumwire import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome(executable_path='chromedriver.exe')
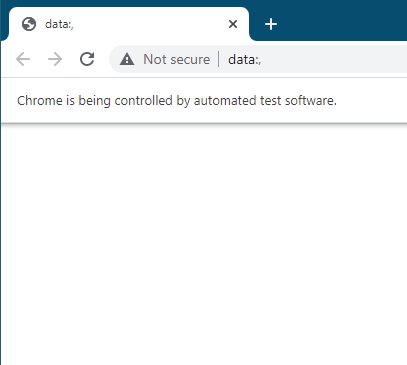
Parsing the website and storing it
We will use the .get() method, to get our page URL, parse it and store it in a variable.
We will use this facebook.com website for our testing purposes.
driver.get(url='https://facebook.com')
Inspecting the webpage for filling
Go to facebook and make sure you are logged out, right click anywhere using chrome browser and click inspect.
Now click on the Email Address field, and you should get the HTML code for it in the inspection widnow. Copy its Full XPATH.
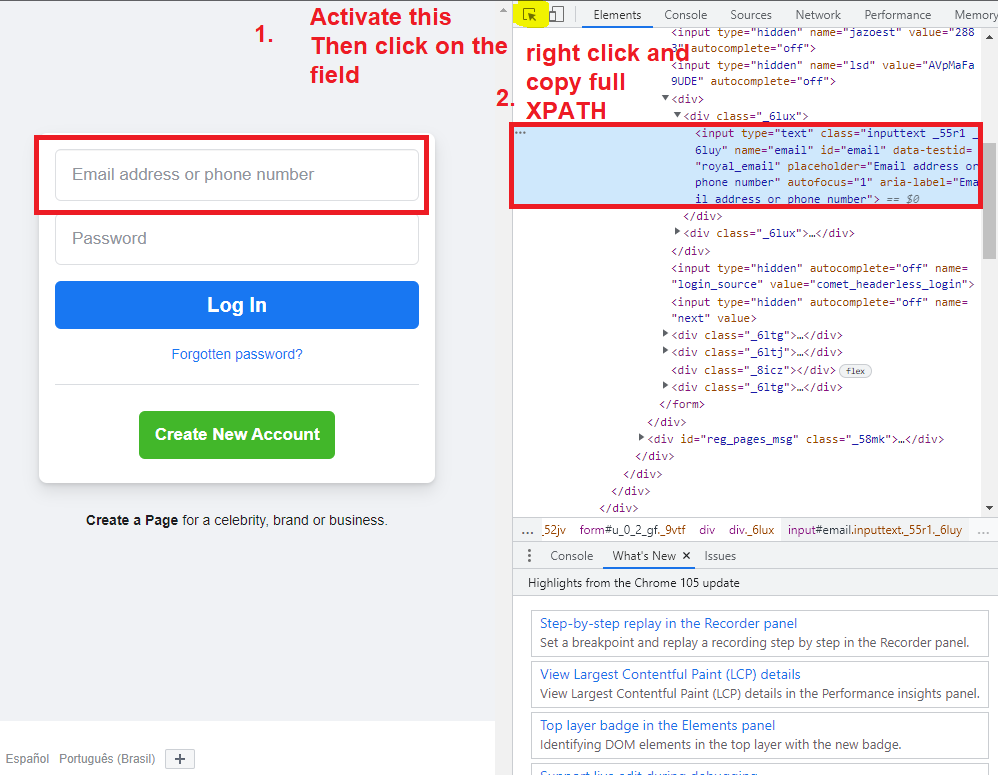
Find element
Since we have parsed the webpage and sored it in driver, now we need to serach for the element we have copied in the parsed version in order to fill the form
We add sleep() function before excuting the next instruction, in order to give time to the program to fill the form.
We do that by calling the methode find_element and the search is done by By.XPATH
And additionally we apply the method send_keys() wand we pass it our email address, which will send it to the facebook email field.
We will repeate the same steps above to get the password field and fill it/>
XPATH_username='/html/body/div[1]/div[1]/div[1]/div/div/div/div[2]/div/div[1]/form/div[1]/div[1]/input'
XPATH_password='/html/body/div[1]/div[1]/div[1]/div/div/div/div[2]/div/div[1]/form/div[1]/div[2]/div/input'
driver.find_element(By.XPATH,XPATH_username).send_keys('exampleEMail@email.com')
sleep(2)
driver.find_element(By.XPATH,XPATH_password).send_keys('passwordOfsomePassword')
sleep(2)
Click
click() is a method we use to click elements on a webpage. We use the same method to get the XPATH of the LogIn button.
driver.find_element(By.XPATH,XPATHClick).click()
sleep(2)
Selenium Web driver Full code
Here is the full code, which will open a facebook page, fill the login info and click on Login Button!
from time import sleep
from seleniumwire import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome(executable_path='chromedriver.exe')
driver.get(url='https://facebook.com')
XPATH_username='/html/body/div[1]/div[1]/div[1]/div/div/div/div[2]/div/div[1]/form/div[1]/div[1]/input'
XPATH_password='/html/body/div[1]/div[1]/div[1]/div/div/div/div[2]/div/div[1]/form/div[1]/div[2]/div/input'
XPATH_click='/html/body/div[1]/div[1]/div[1]/div/div/div/div[2]/div/div[1]/form/div[2]/button'
driver.find_element(By.XPATH,XPATH_username).send_keys('examplEmail@email.com')
sleep(2)
driver.find_element(By.XPATH,XPATH_password).send_keys('passwordOfsomePassword')
sleep(2)
driver.find_element(By.XPATH,XPATH_click).click()
sleep(2)