Automating Sending Emails Using Python and smtplib 2022
We are going to build a python automation mail sending script where are going to use the smtplib library to send the email. Lets dive in!.
Installing secure-smtplib using pip
Use pip to install the library.
pip install secure-smtplib
After installing secure-smtplib we need to import the smtplib in our file and create new two variable one for you email that you want to keep as sender, and other variable is for email's login information (Replace with your own login information, keeping the double quotation marks).
import smtplib
my_gmail ="YOUR_EMAIL_ADDRESS"
my_password ="YOUR_PASSWORD"
The Process of sending Emails:
We will define a new Python Function called sendEmail.
This function will do the following:
- Connect to our target server, in our case Gmail.
- Gmail Server: smtp.gmail.com Gmail Port: 587.
- Start the Authentication Proccess by calling starttls() method.
- Send out our Credentials using login() method.
- Using sendmail() method which will send out our target message to a certain Email Address
And the code should look like this:
def sendMail(reciver,message):
# creting the server connectionof the mail
with smtplib.SMTP('smtp.gmail.com',port=587) as connection:
# supporting the authentication
connection.starttls()
# login to my account
connection.login(user=my_gmail,password=my_password)
# sending the mail
connection.sendmail(from_addr=my_gmail,to_addrs=reciver,msg=f"Subject: Seterlite detected \n\n\n{message}")
Taking user input and calling sendMail() :
Now we can either save our Target email address and message in a seperate variable, or we can let the user type it in. We will go with the later one.
reciver_mail = input("enter the reciver mail:")
message = input("enter the message:")
sendMail(reciver_mail,message)
smtplib Full Code:
Now Let's try running our code:
import smtplib
my_gmail ="YOUR_EMAIL_ADDRESS"
my_password ="YOUR_PASSWORD"
def sendMail(reciver,message):
# creting the server connectionof the mail
with smtplib.SMTP('smtp.gmail.com',port=587) as connection:
# supporting the authentication
connection.starttls()
# login to my account
connection.login(user=my_gmail,password=my_password)
# sending the mail
connection.sendmail(from_addr = my_gmail, to_addrs = reciver,
msg = f"Subject: Seterlite detected \n\n\n{message}")
reciver_mail = input("enter the reciver mail:")
message = input("enter the message:")
sendMail(reciver_mail,message)
Unfortunetly This won't run because, we need to also turn on two-factor verfication on your Gmail account.
Solving smtplib SMTPAuthenticationError issue:
This issue is caused by google security settings. First off, login to your Google Account Settings and choose the security tab.
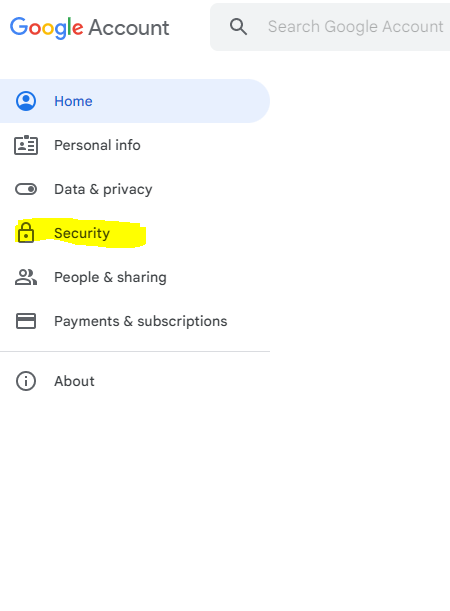
Second Activate two-factor Auth and follow the activation steps.
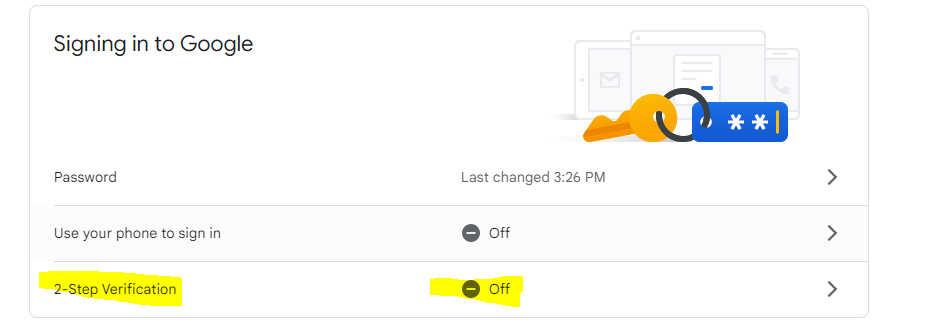
From the same list click on App Passwords
Select your app as Mail, choose your device and click on generate.
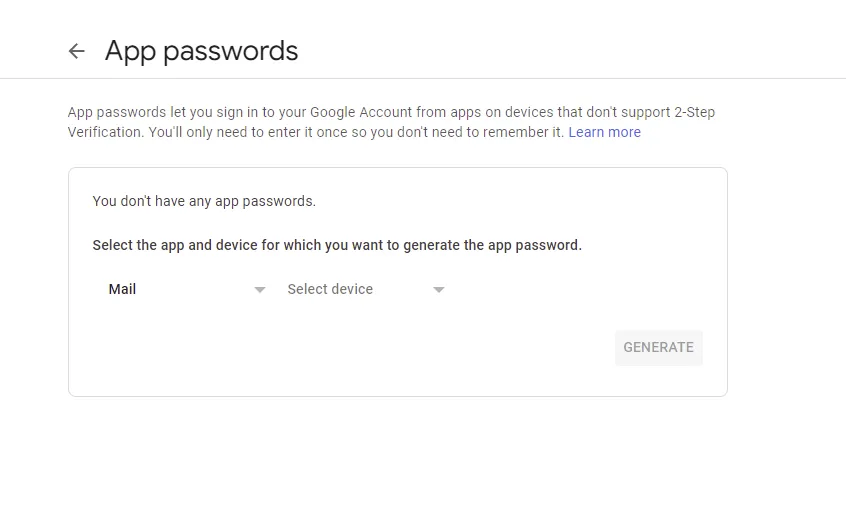
Now rerun your script, check your targe email inbox, and Everything should work out!